Note
This page was generated from a Jupyter notebook. The original can be downloaded from here.
Inactive Unit Analysis: Example¶
This notebook demonstrates how to run IUA on a simple dataset. For more general information about how to use DeepView and about each of these steps, it’s suggested to start with the How-To guides in the docs (starting with how to load a model), and then checking out the Familiarity Notebook for Rare Data and Data Errors.
1. Use DeepView to run inference¶
Let us start by importing everything needed to run on this notebook.
[1]:
from deepview.base import pipeline, ResponseInfo
from deepview.introspectors import IUA
from deepview.samples import StubImageDataset
from deepview.processors import FieldRenamer, Transposer
from deepview.exceptions import enable_deprecation_warnings
enable_deprecation_warnings(error=True) # treat DeepView deprecation warnings as errors
from deepview_tensorflow import load_tf_model_from_path
2025-05-26 20:37:33.218839: E external/local_xla/xla/stream_executor/cuda/cuda_fft.cc:467] Unable to register cuFFT factory: Attempting to register factory for plugin cuFFT when one has already been registered
WARNING: All log messages before absl::InitializeLog() is called are written to STDERR
E0000 00:00:1748291853.233403 6787 cuda_dnn.cc:8579] Unable to register cuDNN factory: Attempting to register factory for plugin cuDNN when one has already been registered
E0000 00:00:1748291853.237670 6787 cuda_blas.cc:1407] Unable to register cuBLAS factory: Attempting to register factory for plugin cuBLAS when one has already been registered
W0000 00:00:1748291853.248888 6787 computation_placer.cc:177] computation placer already registered. Please check linkage and avoid linking the same target more than once.
W0000 00:00:1748291853.248900 6787 computation_placer.cc:177] computation placer already registered. Please check linkage and avoid linking the same target more than once.
W0000 00:00:1748291853.248902 6787 computation_placer.cc:177] computation placer already registered. Please check linkage and avoid linking the same target more than once.
W0000 00:00:1748291853.248904 6787 computation_placer.cc:177] computation placer already registered. Please check linkage and avoid linking the same target more than once.
2025-05-26 20:37:33.252833: I tensorflow/core/platform/cpu_feature_guard.cc:210] This TensorFlow binary is optimized to use available CPU instructions in performance-critical operations.
To enable the following instructions: AVX2 FMA, in other operations, rebuild TensorFlow with the appropriate compiler flags.
Download a model, MobileNet, and store it locally¶
[2]:
from deepview_tensorflow import TFModelExamples
mobilenet = TFModelExamples.MobileNet()
model = mobilenet.model
2025-05-26 20:37:34.947769: E external/local_xla/xla/stream_executor/cuda/cuda_platform.cc:51] failed call to cuInit: INTERNAL: CUDA error: Failed call to cuInit: UNKNOWN ERROR (303)
Create a Producer to generate data¶
[3]:
data_producer = StubImageDataset(
dataset_size=32,
image_width=224,
image_height=224,
channel_count=3
)
Find Convolutional Layers¶
[4]:
conv2d_responses = [
info.name
for info in model.response_infos.values()
if info.layer.kind is ResponseInfo.LayerKind.CONV_2D
]
Set up processing pipeline¶
[5]:
response_producer = pipeline(
data_producer,
FieldRenamer({"images": "input_1:0"}),
model(conv2d_responses),
Transposer(dim=(0, 3, 1, 2))
)
2. Execute IUA introspector¶
Which can be done with just a single line of code!
[6]:
iua = IUA.introspect(response_producer)
/opt/hostedtoolcache/Python/3.10.17/x64/lib/python3.10/site-packages/keras/src/models/functional.py:241: UserWarning: The structure of `inputs` doesn't match the expected structure.
Expected: [['input_layer']]
Received: inputs=['Tensor(shape=(32, 224, 224, 3))']
warnings.warn(msg)
Show table of results¶
IUA.show(iua)
will show, by default, a table of layers and the discovered inactive units.
[7]:
print(IUA.show(iua).head())
response mean inactive std inactive
0 conv1 100352.59375 0.654760
1 conv1_bn 0.00000 0.000000
2 conv1_relu 122898.53125 204.862146
3 conv_dw_1 87842.87500 8.184398
4 conv_dw_10 23058.00000 408.534041
Plot results¶
IUA.show
can also be used to view charts, by setting vis_type
to IUA.VisType.CHART
.
One layer’s chart can be viewed in this manner, e.g. conv_pw_9
…
[8]:
IUA.show(iua, vis_type=IUA.VisType.CHART, response_names=['conv_pw_9'])
[8]:
<Axes: title={'center': 'conv_pw_9 Inactive Unit Proportions '}>
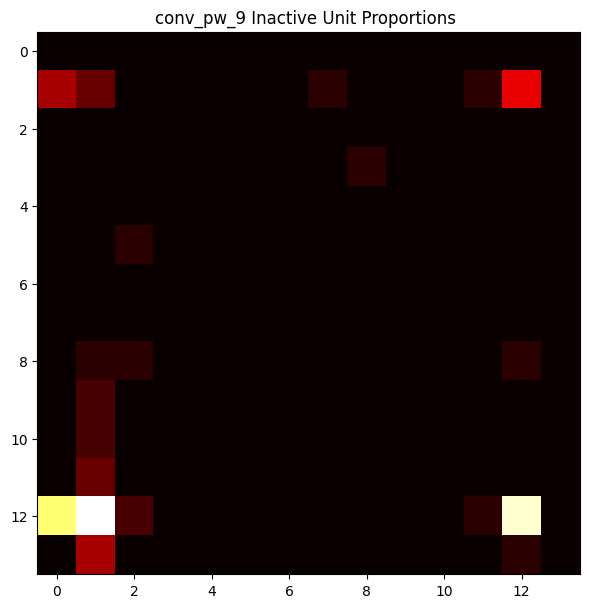
… or view all responses’ charts (omitting the response_names
parameter):
[9]:
IUA.show(iua, vis_type=IUA.VisType.CHART)
[9]:
array([<Axes: title={'center': 'conv1 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv1_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv1_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_1 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_10 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_10_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_dw_10_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_11 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_11_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_dw_11_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_12 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_12_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_dw_12_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_13 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_13_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_dw_13_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_1_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_dw_1_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_2 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_2_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_dw_2_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_3 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_3_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_dw_3_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_4 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_4_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_dw_4_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_5 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_5_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_dw_5_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_6 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_6_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_dw_6_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_7 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_7_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_dw_7_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_8 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_8_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_dw_8_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_9 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_dw_9_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_dw_9_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pad_12 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pad_2 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pad_4 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pad_6 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_preds Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_1 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_10 Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_10_bn Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_10_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_11 Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_11_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_11_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_12 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_12_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_12_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_13 Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_13_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_13_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_1_bn Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_1_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_2 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_2_bn Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_2_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_3 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_3_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_3_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_4 Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_4_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_4_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_5 Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_5_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_5_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_6 Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_6_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_6_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_7 Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_7_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_7_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_8 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_8_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_8_relu Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_9 Inactive Unit Proportions '}>,
<Axes: title={'center': 'conv_pw_9_bn Inactive Unit Proportions - no inactive units'}>,
<Axes: title={'center': 'conv_pw_9_relu Inactive Unit Proportions '}>],
dtype=object)

[ ]: